I have a blog again, hello. I thought a first reasonable thing to talk about would be this very website. Specifically, the page transitions. The system behind these page transitions actually took an unreasonable amount of work to get to a reliable and controllable state. It's worth noting that the system came about when tasked with recreating the page transitions for michaellawrence.studio originally created by Piers Olenski. The website was built with Gatsby and was being ported to NextJS and so the page transitions needed to be recreated. Gatsby had a pretty amazing plugin called gatsby-plugin-transition-link which did an amazing job of handling reasonably complex page transitions, but was made specifically for the Gatsby router.
We also had the intention of moving over the Framer Motion as it is newer and flashier, and has layout animations which should make this kind of thing a piece of cake. It turned out to be an absolute nightmare and a huge amount of work to find out that it just wasn't going to work - honestly for reasons I can't remember now, I just know it took me well over a week of work of trial and error and many demo apps to figure out Framer just wasn't up for this specific task.
The original implementation would intercept the router at the point of clicking on a link and hold the outgoing page and the incoming page in a transitonal time. During that moment you have access to both tree's of components and you can animate them as you wish, before finally unmounting the outgoing and leaving the incoming mounted.
To acheive this in Next I took the approach of Olivier Larose where we wrap <Component />
in a transition layer which handles the rendering of the the <Component />
itself, shown below:
export default function App({ Component, router }: AppProps) {
return (
...
<TransitionProvider>
<Transition>
<Component key={router.asPath} {...pageProps} />
</Transition>
</TransitionProvider>
...
)
}
The crux of it is as follows:
export const Transition = ({ children }: any) => {
const [exitChild, setExitChild] = useState(children);
const [enterChild, setEnterChild] = useState(null);
useEffect(() => {
if (children.key === exitChild.key) return;
/* Mount new child. It is initially hidden by the current
* exit child providing a background color is set. */
setEnterChild(children);
/* Do fun stuff */
animate();
/* ...and then eventually */
setExitChild(null);
}, [children, exitChild.key, animate]);
return (
<>
<TransitionCloneContainer ref={overlayRef} />
{enterChild}
{exitChild}
</>
);
};
children
in this case is the page <Component />
which a prop to our <Transition />
component. We detect prop change by passing in, and keeping an eye on children.key
. We are also using useState
to control what gets returned and rendered from <Transition />
, so given that we have both the incoming and outgoing page components, this is when we can play with the transition.
The page transitions we're after is to have elements of the previous page animate to the exact position of the (seemingly) same element on the new page. The challenge however is how to keep track of what we want to animate in a React-y way. The previous Gatsby implementation used typical DOM selectors, which isn't a bad thing, but it's kinda seen as an anti-pattern in React as in theory React is handling the entire tree of elements in the DOM at any given type, so using DOM selectors works outside of that system and is a gateway to side-effects and so is very non-React. It's up to you how my you care about that, but for this we wanted to keep track of elements using refs
, the React way.
A ref
should be created when a component mounts, so already means no traversal of the DOM tree (as is the DOM selector way). So that then means we need to declare what should be transisions when it mounts. This led to a context layer and the <TransitionProvider />
. The <TransitionProvider />
essentially just stores a map of pagePath -> elements-to-morph
. We'll go into how in a sec, but given this happens on mount, there is a moment before the exiting page is unmounting that the map will have:
{
oldPage -> oldPageElements,
newPage -> newPageElements
}
So with this we can then check which old elementing is animating to which new element.
How
The <TransitionProvider />
exports two functions for handling the refsMap
. I won't go into every detail here but it exports a register
and unregister
function which just adds or removes items from the component store. A HTMLElement
in this context is a React ref
:
export type MorphItems = Map<string, HTMLElement>;
type ComponentStoreData = {
page?: HTMLElement;
morphItems: MorphItems;
};
type ComponentStore = Map<Path, ComponentStoreData>;
Again, I won't go into the details but another hook (useContextRef
) provides two helper functions which wrap the above functions so they are a bit more context aware. These can then be used like so:
export default function Home() {
const pathname = useCachedPathname();
const { setMorphItem } = useContextRef(pathname);
return (
<Page>
<Main>
<H1 ref={setMorphItem<HTMLHeadingElement>("josh-murr")}>Josh Murr</H1>
</Main>
</Page>
);
}
As an aside, we have to cache the pathname using useState
to hold the outgoing and incoming pathnames in state simultaneously.
It can be a bit confusing to use, but any where that the key from one morph item matches the key of another morph item on another page, that item will get morph'd. So it's best to use generated keys from the text content or something similar rather than explicit keys if you want something like a nav item to animate from one page to any other page.
Animations
Finally we use GSAP to do the actual animations. Again, no details here, but the general orchestration is as follows:
- Capture in the incoming
children
prop (the incoming page). - Place this in the DOM underneath the outgoing (using
useState
). - Iterate over the incoming and outgoing refs from the component store and: clone the outgoing node and get the target position from the incoming node.
- Fade out the exiting page.
- Animate the cloned node to the target position.
- Fade in the incoming page.
- Remove all the cloned nodes.
- Remove all the cloned nodes.
- Remove all the cloned nodes.
- Remove all the cloned nodes.
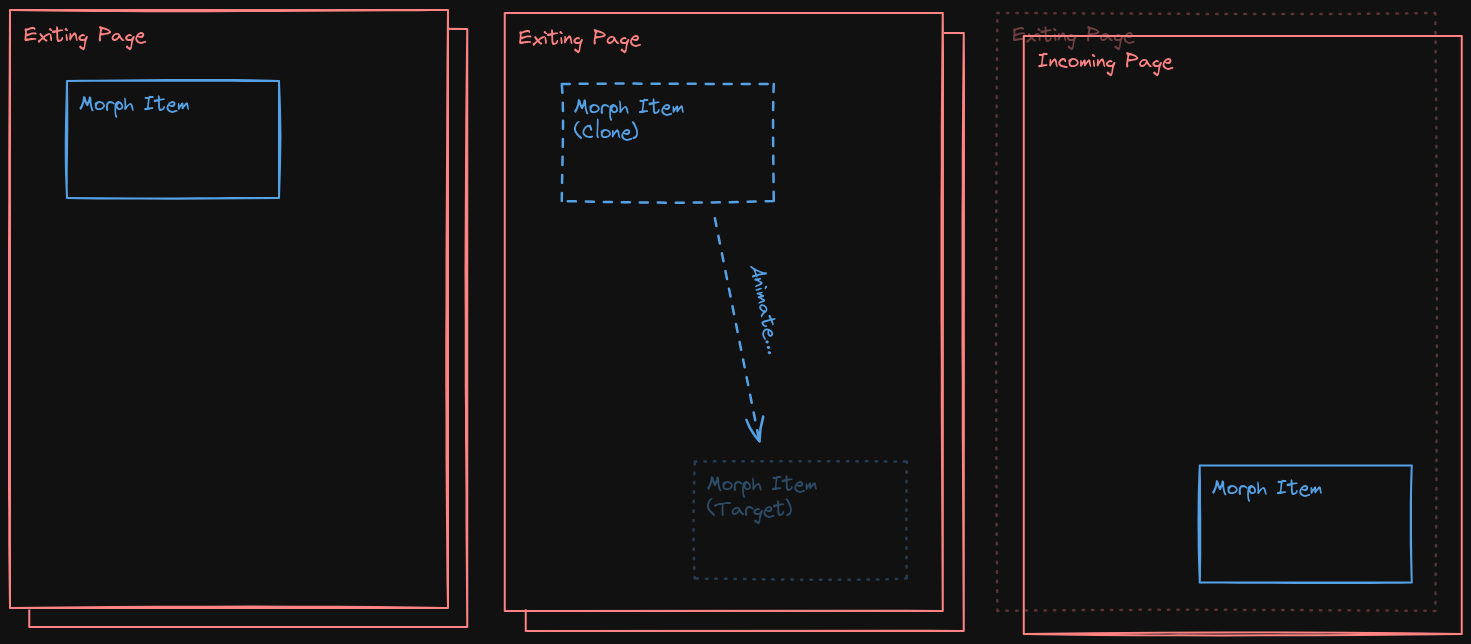
And Bob's yer uncle! As I said it took quite a long time to get to this point, but now it is a pretty flexible and reasonably robust system. It's tailor made for the NextJS Pages router at this point; I've thought about extracting it out as it's own package, and even abstracting out the animations so it's not dependant on GSAP, but that's quite low on my TODO list right now. Maybe one day.
You can look at the code for this site here.